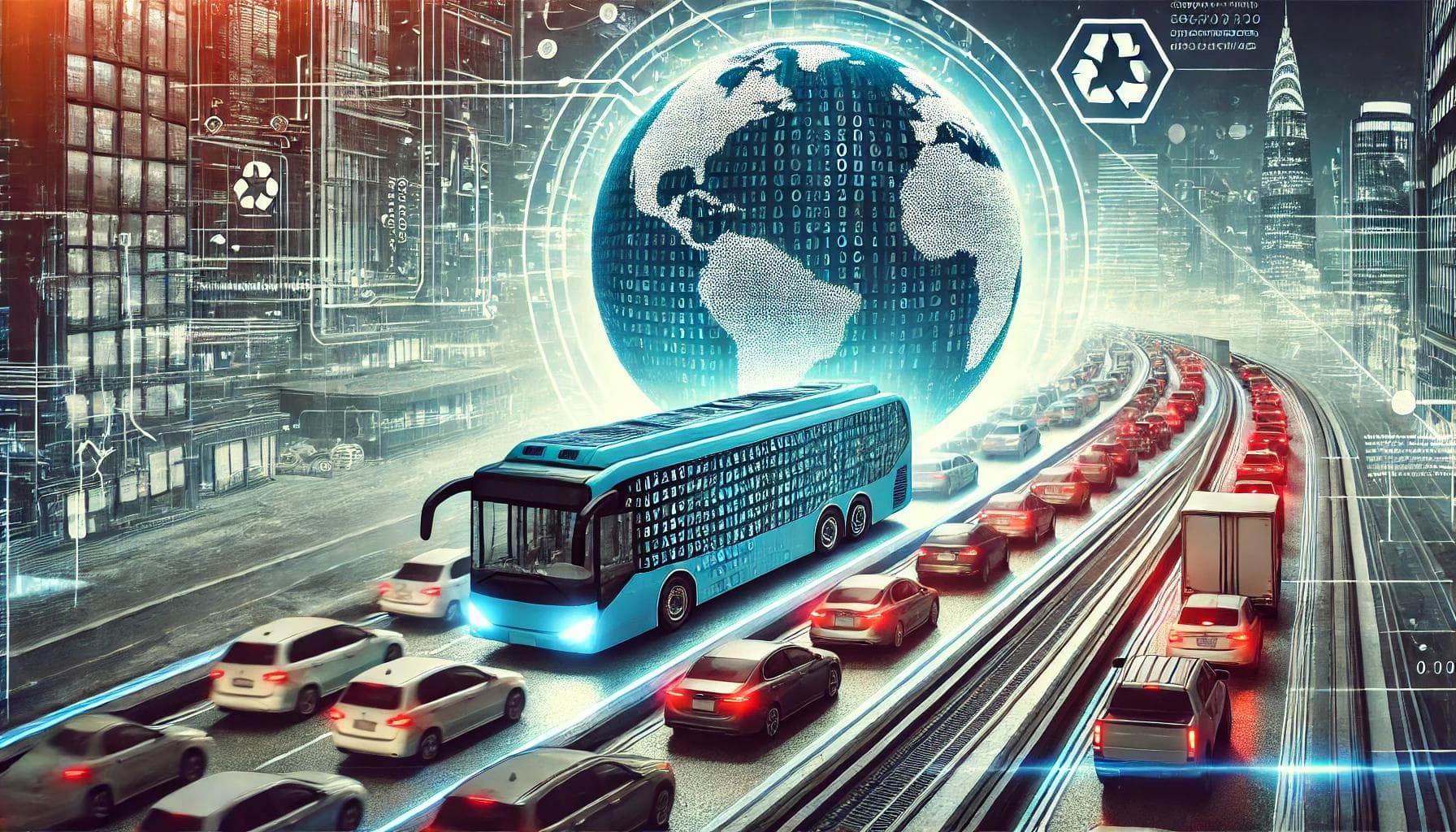
The Evolution of Global Variables: From Assembly to Modern State Management
July 26, 2024
The Evolution of Global Variables: From Assembly to Modern State Management
In the ever-evolving world of software development, global variables have transformed significantly. From their humble beginnings as simple memory locations in assembly language, they’ve become the sophisticated state management solutions we rely on today. This journey isn’t just about technological advancements; it’s about improving how we write, manage, and maintain code.
From Assembly to C: The Wild West
Back in the early days of programming, assembly language was the sheriff in town. Global variables were just memory addresses any part of the program could access. It was like the Wild West—simple and effective for small programs but chaotic as software complexity grew.
Global Variable in Assembly:
section .data
global_var db 10
This simplicity had a downside. With no data encapsulation, any part of the program could change the global variable, leading to unintended consequences and making debugging a headache.
When high-level languages like C came along, they brought some order. Global variables were still a thing, but at least there was some structure.
Global Variable in C:
#include <stdio.h>
int global_var = 10;
void printGlobal() {
printf("Global variable: %d\n", global_var);
}
int main() {
printGlobal();
return 0;
}
Despite the improvements, global variables in C still had issues with encapsulation, testing, and maintainability.
The OOP Revolution: Java Steps In
The shift to Object-Oriented Programming (OOP) was a game-changer. Java, with its focus on encapsulation, inheritance, and polymorphism, offered a more organized way to handle state.
Static Variable in Java:
public class MyClass {
private static int globalVar = 10;
public static void printGlobalVar() {
System.out.println("Global variable: " + globalVar);
}
}
In Java, static
variables allowed shared state across instances while keeping things tidy. This approach reduced the risk of unexpected changes, but too many static variables could still cause problems similar to old-school global variables.
The Web Era: Rise of Event Buses
As web technologies advanced, the need for efficient state management grew. Event-driven programming emerged as a powerful way to handle the complexity of modern web apps. Event buses let different parts of an application communicate by sending and receiving events.
Event Bus in Node.js:
const EventEmitter = require('events');
const eventEmitter = new EventEmitter();
eventEmitter.on('event', () => {
console.log('Event triggered!');
});
eventEmitter.emit('event');
Event buses decoupled components, making the code more modular and easier to maintain. This approach was especially useful for handling asynchronous operations and user interactions in web apps.
Modern State Management: Redux and Beyond
As web apps got more complex, developers needed better state management solutions. Enter libraries like Redux and Vuex, bringing a new level of sophistication to state management in JavaScript.
State Management with Redux:
import { createStore } from 'redux';
const initialState = {
globalVar: 10
};
function reducer(state = initialState, action) {
switch (action.type) {
case 'UPDATE_VAR':
return { ...state, globalVar: action.payload };
default:
return state;
}
}
const store = createStore(reducer);
store.dispatch({ type: 'UPDATE_VAR', payload: 20 });
console.log(store.getState());
Redux and similar libraries introduced the concept of a single source of truth, making state transitions predictable and debugging easier. Centralizing state management improved code maintainability and scalability, addressing many issues tied to traditional global variables.
What now?
The evolution of global variables—from simple memory locations to modern state management solutions—shows how far we’ve come. As apps get more complex, the need for better(and simpler to handle) state management will keep growing. Understanding this helps us build better, more maintainable software. From assembly to modern state management, the code scope isn't losing its charm for new ideas.